Note, this article is not finished! You can help by editing this doc page.
Overview
- Simplified
- Detailed
template< class CharT, /* ... */ >
class basic_string;
- Regular
- Polymorphic (since C++17)
template<
class CharT,
class Traits = std::char_traits<CharT>,
class Allocator = std::allocator<CharT>
>
class basic_string;
namespace pmr {
template< class CharT, class Traits = std::char_traits<CharT> >
using basic_string = std::basic_string<CharT, Traits,
std::polymorphic_allocator<CharT>>;
}
A class that is used to store and manipulate sequence of characters.
Memory
The elements of a string are stored contiguously in memory.
This means that a pointer to an element of a string
may be passed to any function
that expects a pointer to an element of an array of characters.
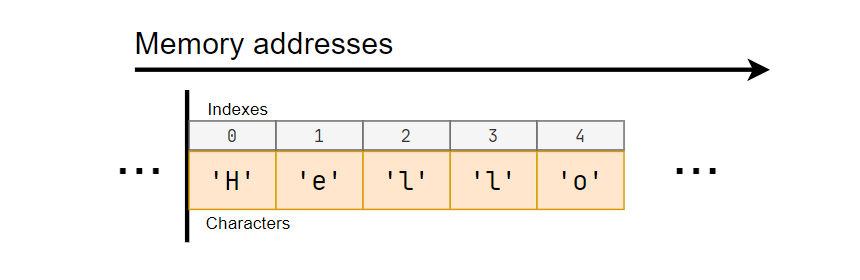
Aliases
Type std::string
is in fact an alias to: std::basic_string<char>
.
Available std::basic_string
aliases
pub | std::string | std::basic_string<char> |
pub | std::wstring | std::basic_string<wchar_t> |
pub | std::u8string (since C++20) | std::basic_string<char8_t> |
pub | std::u16string (since C++11) | std::basic_string<char16_t> |
pub | std::u32string (since C++11) | std::basic_string<char32_t> |
pub | std::pmr::string (since C++17) | std::pmr::basic_string<char> |
pub | std::pmr::wstring (since C++17) | std::pmr::basic_string<wchar_t> |
pub | std::pmr::u8string (since C++20) | std::pmr::basic_string<char8_t> |
pub | std::pmr::u16string (since C++17) | std::pmr::basic_string<char16_t> |
pub | std::pmr::u32string (since C++17) | std::pmr::basic_string<char32_t> |
Technical details
Technical definition of a string
The class template basic_string
stores and manipulates sequences of char
-like objects,
which are non-array objects of trivial
standard-layout type. The class is dependent neither
on the character type nor on the nature of operations on that type.
The definitions of the operations are supplied via the Traits
template parameter - a specialization
of std::char_traits
or a compatible traits class. Traits::char_type
and CharT
must name the same type; otherwise the program is ill-formed
The elements of a basic_string are stored contiguously, that is, for a basic_string
s,
&*(s.begin() + n) == &*s.begin() + n
for any n in [ 0, s.size() ), or, equivalently,
a pointer to s[0]
can be passed to functions that expect a pointer to the first element
of a null-terminated (since C++11) CharT[]
array.
std::basic_string
Defined in | string |
Template parameters
pub | CharT | A character type. |
pub | Traits | Traits class specifying the operations on the character type. |
pub | Allocator | Allocator type used to allocate internal storage. |
Type names
pub | traits_type | Traits |
pub | value_type | CharT |
pub | allocator_type | Allocator |
pub | size_type | Allocator::size_type (until C++11) std::allocator_traits<Allocator>::size_type (since C++11) |
pub | difference_type | Allocator::difference_type (until C++11) std::allocator_traits<Allocator>::difference_type (since C++11) |
pub | reference | value_type& |
pub | const_reference | value_type const& |
pub | pointer | Allocator::pointer (until C++11) std::allocator_traits<Allocator>::pointer (since C++11) |
pub | const_pointer | Allocator::const_pointer (until C++11) std::allocator_traits<Allocator>::const_pointer (since C++11) |
pub | iterator |
|
pub | reverse_iterator | std::reverse_iterator<iterator> |
pub | const_reverse_iterator | std::reverse_iterator<const_iterator> |
Member functions
pub | (constructors) | Constructs a |
pub | (destructor) | Destroys the string, deallocating internal storage if used. |
pub | operator= | Assigns values to the string. |
pub | assign | Assigns characters to the string. |
pub | get_allocator | Returns the associated allocator. |
Element access
pub | at | Accesses the specified character with bounds checking. |
pub | operator[] | Accesses the specified character. |
pub | front (since C++11) | Returns the first character. |
pub | back (since C++11) | Returns the last character. |
pub | data | Returns a pointer to the first character of a string. |
pub | c_str | Returns a non-modifiable standard C character array version of the string. |
pub | operator basic_string_view (since C++17) | Returns a non-modifiable |
Iterators
pub | begin cbegin (since C++11) | Returns an |
pub | end cend (since C++11) | Returns an |
pub | rbegin crbegin (since C++11) | Returns an reverse |
pub | rend crend (since C++11) | Returns an reverse |
Capacity
pub | empty | Returns |
pub | size length | Returns the number of characters. |
pub | max_size | Returns the maximum number of characters. |
pub | reserve | Reserves storage |
pub | capacity | Returns the number of characters that can be held in currently allocated storage |
pub | shrink_to_fit (since C++11) | Reduces memory usage by freeing unused memory. |
Operations
pub | clear | Clears the contents. |
pub | insert | Inserts characters. |
pub | erase | Removes characters. |
pub | push_back | Appends a character to the end. |
pub | pop_back (since C++11) | Removes the last character. |
pub | append | Appends characters to the end. |
pub | operator+= | Appends characters to the end. |
pub | compare | Compares two strings. |
pub | starts_with (since C++20) | Checks if the string starts with the given prefix. |
pub | ends_with (since C++20) | Checks if the string ends with the given suffix. |
pub | contains (since C++23) | Checks if the string contains the given substring or character. |
pub | replace | Replaces specified portion of a string. |
pub | substr | Returns a substring. |
pub | copy | Copies characters. |
pub | resize | Changes the number of characters stored. |
pub | resize_and_overwrite (since C++23) | Changes the number of characters stored and possibly overwrites indeterminate contents via user-provided operation. |
pub | swap | Swaps the contents. |
Search
pub | find | Find characters in the string. |
pub | rfind | Find the last occurrence of a substring. |
pub | find_first_of | Find first occurrence of characters. |
pub | find_first_not_of | Find first absence of characters. |
pub | find_last_of | Find last occurrence of characters. |
pub | find_last_not_of | Find last absence of characters. |
Constants
pubstaticconstexpr | npos | A special value. The exact meaning depends on the context. |
Non-member functions
pub | operator+ | Concatenates two strings or a string and a char. |
pub | operator== operator!= (removed in C++20) operator< (removed in C++20) operator> (removed in C++20) operator<= (removed in C++20) operator>= (removed in C++20) operator<=> | Lexicographically compares two strings. |
pub | std::swap (std::basic_string) | An overload for a std::swap algorithm. |
pub | erase (std::basic_string) erase_if (std::basic_string) | Overloads for std::erase/std::erase_if algorithms. |
Input/output
pub | operator<< operator>> | Performs stream input and output on strings. |
pub | getline | Read data from an I/O stream into a string. |
Numeric conversions
pub | stoi stol stoll | Converts a string to a signed integer. |
pub | stoui stoul stoull | Converts a string to an unsigned integer. |
pub | stof stod stold | Converts a string to a floating point value. |
pub | to_string | Converts an integral or floating point value to |
pub | to_wstring | Converts an integral or floating point value to |
Literals
Defined in namespacestd::literals::string_literals
pub | operator ""s | Converts a character array literal to |
Helper classes
pub | std::hash<std::string> (since C++11) std::hash<std::u8string> (since C++20) std::hash<std::u16string> (since C++11) std::hash<std::u32string> (since C++11) std::hash<std::wstring> (since C++11) std::hash<std::pmr::string> (since C++17) std::hash<std::pmr::u8string> (since C++20) std::hash<std::pmr::u16string> (since C++17) std::hash<std::pmr::u32string> (since C++17) std::hash<std::pmr::wstring> (since C++17) | Specializations for |
Deduction guides (since C++17)
Click to expand
Deduction guides
// (1)
template< class InputIt, class Alloc = std::allocator<
typename std::iterator_traits<InputIt>::value_type> >
basic_string( InputIt, InputIt, Alloc = Alloc() )
-> basic_string<typename std::iterator_traits<InputIt>::value_type,
std::char_traits<typename std::iterator_traits<InputIt>::value_type>,
Alloc>;
(1)
allows deduction from a iterator range
// (2)
template< class CharT,
class Traits,
class Alloc = std::allocator<CharT> >
explicit basic_string( std::basic_string_view<CharT, Traits>, const Alloc& = Alloc() )
-> basic_string<CharT, Traits, Alloc>;
// (3)
template< class CharT,
class Traits,
class Alloc = std::allocator<CharT>> >
basic_string( std::basic_string_view<CharT, Traits>, typename /*see below*/::size_type,
typename /*see below*/::size_type, const Alloc& = Alloc() )
-> basic_string<CharT, Traits, Alloc>;
(2)
and(3)
allow deduction from astd::basic_string_view<CharT, Traits>
The size_type
parameter type refers to the size_type
member type of the type deduced by the deduction guide.
Overload resolution
In order for any of the deduction guides to participate in overload resolution, the folllowing requirements must be met:
- for
(1)
,InputIt
satisfiesLegacyInputIterator
- for
(1 - 3)
,Alloc
satisfiesAllocator
The extent to which the library determines that a type does not satisfy LegacyInputIterator
is unspecified,
except that as a minimum:
- Integral types do not qualify as input iterators.
Likewise, the extent to which it determines that a type does not satisfy Allocator
is unspecified,
except that as a minimum:
- The member type
Alloc::value_type
must exist. - The expression
std::declval<Alloc&>().allocate(std::size_t{})
must be well-formed when treated as an unevaluated operand.
Examples
Basic manipulation
#include <iostream>
#include <string>
int main()
{
std::string s = "World";
std::cout << "Hello, " << s << "!" << std::endl;
}
Hello, World!
#include <iostream>
#include <string>
int main()
{
std::string a = "Hello, ";
std::string b = "World!";
std::string c = a + b;
std::cout << c;
}
Hello, World!
#include <iostream>
#include <string>
int main()
{
// Split by the comma.
// v
std::string a = "Hello, World!";
size_t pos = a.find(',');
std::string hello = a.substr(0, pos);
// 1 for a comma, and 1 for a space before "World"
std::string world = a.substr(pos + 1 + 1);
std::cout << hello << '\n' << world;
}
Hello
World!
Conversions
#include <iostream>
#include <string>
int main()
{
std::string numberInString = "8314";
// Note: stoi can throw exception if failed!
int number = std::stoi(numberInString);
std::cout << "Number: " << number;
}
Number: 8314
#include <iostream>
#include <string>
int main()
{
std::string numberInString = "3.141";
// Note: stof can throw exception if failed!
float number = std::stof(numberInString);
std::cout << "Number: " << number;
}
Number: 3.141
Convert numbers to std::string
#include <iostream>
#include <string>
int main()
{
std::string numberInString = "3.141";
// Note: stof can throw exception if failed!
float number = std::stof(numberInString);
std::cout << "Number: " << number;
}
s1: 123
s2: 456.200012
s3: 3.141590
If you want a custom formatting, use std::stringstream
,
or a non-standard library fmtlib.
Hover to see the original license.